
Lately on one of my projects I had introduce many webservices that uses function modules to process data through BAPI which we also had to extensively test and that’s when a question arise:
Is there a way to automatically copy data to function module that's being called from webservice as the test data?
Till now we always did it manually and actually I had thought about it a few times in the past, but whenever I started searching for the solution nothing turned up. Now since I had a bit of time I decided to do some more digging and find an optimal solution to the problem.
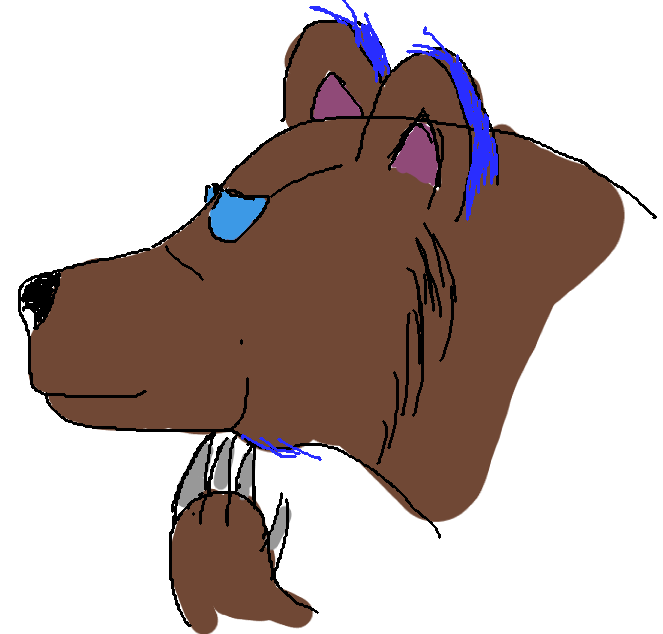
As I already wrote I first went to look for a standard solution. I looked not only through the SAP notes but also the blogs and other available mediums. However even though the problem popped out a few times I saw only one resolution to it, which was used.
If you can trigger the ABAP proxy on the SAP side or catch the communicate live you can always save the data from the debugger view by simply clicking the following buttons. It’s the easiest way by far and can be used not only for the XML documents, but when testing every FM in every possible scenario.
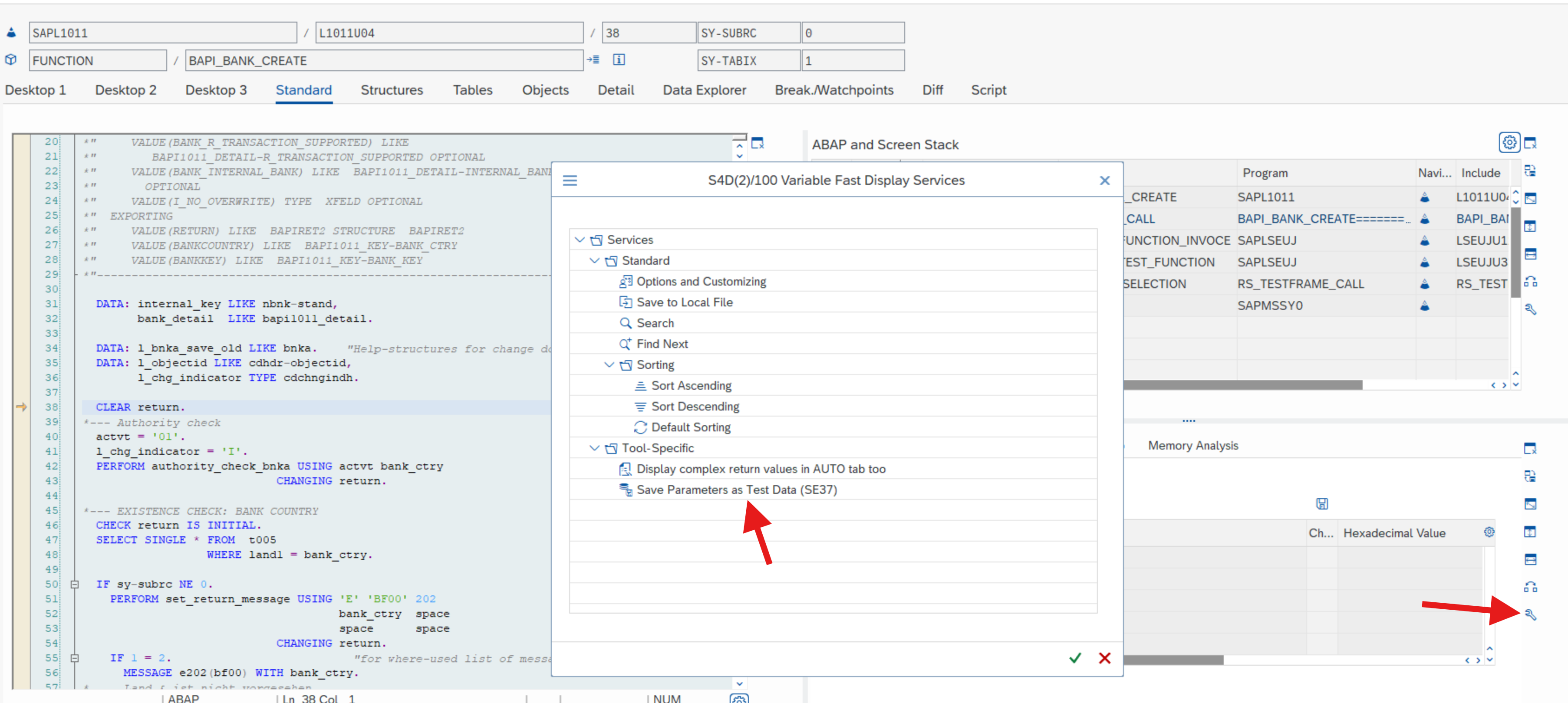
However what if you can’t do either of those? If you have only permission to selected objects and the abap proxy is not in them and also there is no possibility to send it and catch live? Somehow in this scenario if you can create a new function module or program you might still be able to save some time. My first thought about solution for this problem was that we should be able to create a program that can simply create a “test data” just like we would do it manually. However I couldn’t find any bapi/FM/program that we could run to do it, so I tried to go around problem. If we will be running the program ourselves we could just read the XML and call the function module we want next. Then we can just use the solution I mentioned earlier and save the data during the debugger. If take for example following XML that we want to test:
<?xml version="1.0" encoding="UTF-8"?>
<company>
<employee>
<name>John Doe</name>
<title>Software Engineer</title>
<department>Development</department>
</employee>
<employee>
<name>Jane Smith</name>
<title>Project Manager</title>
<department>Management</department>
</employee>
</company>
The program to parse it and call another FM would look as following
DATA: lt_xml_data TYPE TABLE OF string,
lt_employees TYPE TABLE OF ty_employee,
lv_xml_line TYPE string,
lv_file_path TYPE string.
FIELD-SYMBOLS: <fs_employee> TYPE ty_employee.
* Define structure for employee data
TYPES: BEGIN OF ty_employee,
name TYPE string,
title TYPE string,
department TYPE string,
END OF ty_employee.
* Prompt user to select XML file
CALL FUNCTION 'F4_FILENAME'
EXPORTING
program_name = sy-repid
dynpro_number = sy-dynnr
field_name = 'lv_file_path'
IMPORTING
file_name = lv_file_path.
IF sy-subrc <> 0.
WRITE: / 'Error selecting file'.
EXIT.
ENDIF.
* Upload XML file
CALL FUNCTION 'GUI_UPLOAD'
EXPORTING
filename = lv_file_path
filetype = 'ASC'
TABLES
data_tab = lt_xml_data
EXCEPTIONS
file_open_error = 1
file_read_error = 2
no_batch = 3
gui_refuse_filetransfer = 4
invalid_type = 5
OTHERS = 6.
IF sy-subrc <> 0.
WRITE: / 'Error uploading file'.
EXIT.
ENDIF.
* Parse XML data
CALL TRANSFORMATION id
SOURCE XML lt_xml_data
RESULT XML lv_xml_line.
* Read XML data into internal table
CALL TRANSFORMATION zxml_to_itab
SOURCE XML lv_xml_line
RESULT employees = lt_employees.
* Call the next function module
CALL FUNCTION 'NEXT_FUNCTION_MODULE'
TABLES
employees = lt_employees.
Of course we also need to create the transformation for it to work, which would look like this
<?xml version="1.0" encoding="UTF-8"?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:output method="xml" indent="yes"/>
<xsl:template match="/">
<tt:root xmlns:tt="http://www.sap.com/transformation-templates">
<tt:employees>
<xsl:for-each select="company/employee">
<tt:employee>
<tt:name>
<xsl:value-of select="name"/>
</tt:name>
<tt:title>
<xsl:value-of select="title"/>
</tt:title>
<tt:department>
<xsl:value-of select="department"/>
</tt:department>
</tt:employee>
</xsl:for-each>
</tt:employees>
</tt:root>
</xsl:template>
</xsl:stylesheet>
With this we would be able to run our desired function module and save the data. Of course this solution is as problematic as the XML and its transformation is. Fortunately in todays world, we would be able to use the AI, to create it for us by simply providing the XML in the prompt. After creating a few of those in the old days and testing them through the whole nights I would rather do anything else than do it again. So are those all of the possibilities for us?
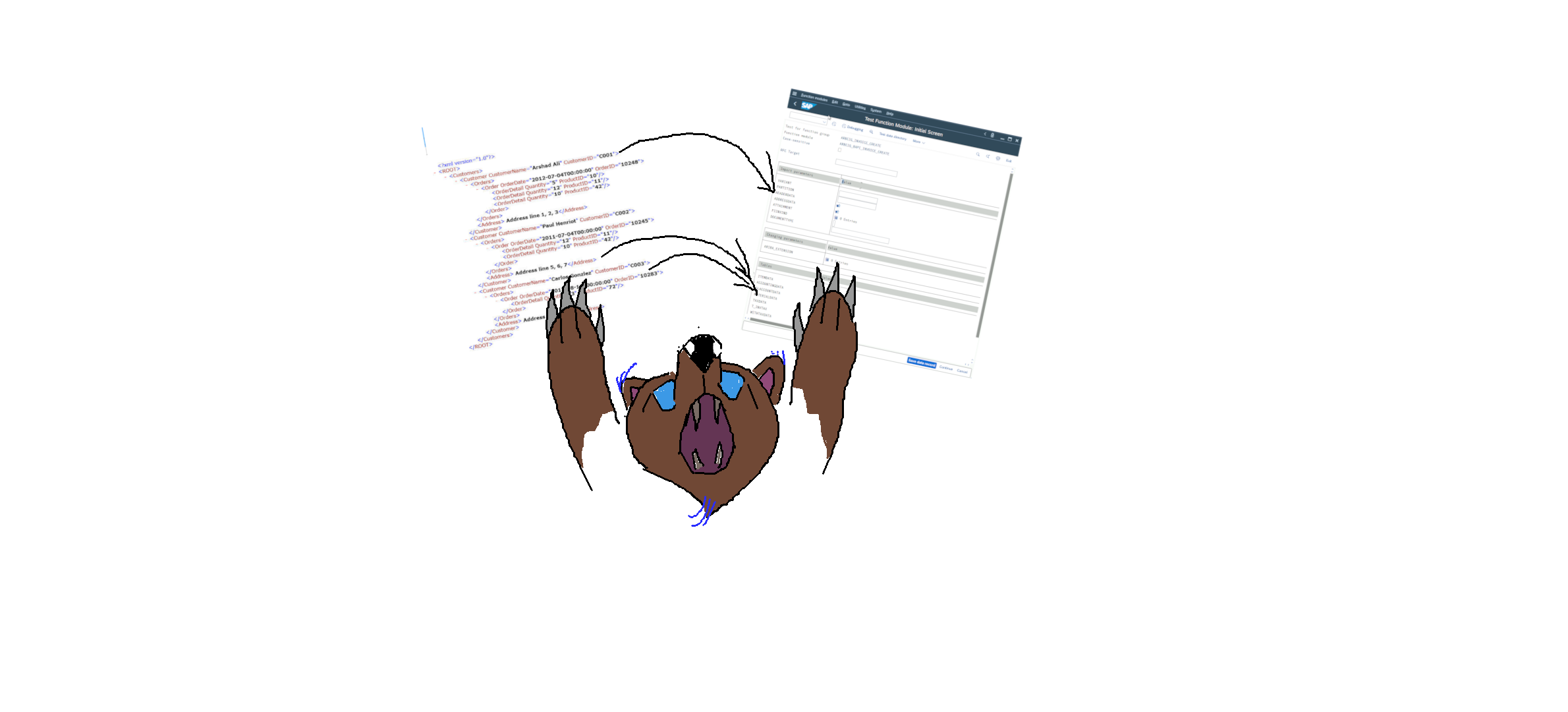
No, in the end I found out that there is one more path we could take, if you can modify the function module you want to test and that is report FBGENMAC (1). The report is self explanatory, you simply provide the name of function module and mode in which it should be done (usually you want “B”).
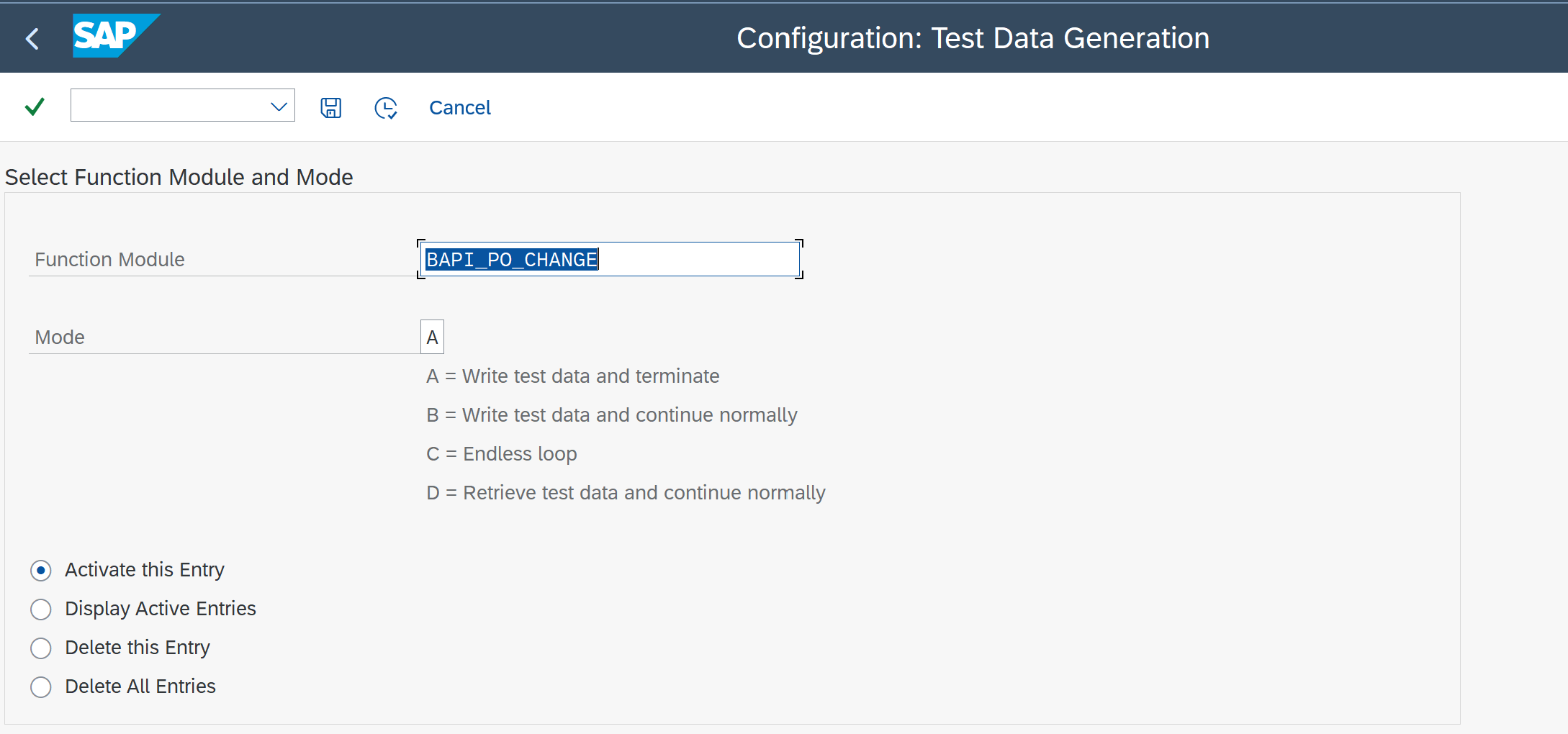
Afterwards if you have the parameter FBGENMAC on the user that will execute your FM and the FM itself has the following lines of code
INCLUDE FBGENMAC.
SET EXTENDED CHECK OFF.
fbgenmac '<<YOUR_FM_NAME>>'.
SET EXTENDED CHECK ON.
It will generate the test data with every run as follows:

This way we can create big amount of test data if we can’t reach the 3rd party team which would provide us the xml or shoot the data to catch it live. Just remember to switch the configuration off once you have what you want, so the test data directory won’t be flooded with generated test scenarios.
Of course one could dream of another scenario and that is marrying the second solution of reading xml from the user PC and running the FM with the FBGENMAC functionality, however that would more abap work without any feasible profits, so I won’t even try it.
Conclusion:
Since we were looking for a automatic way to create test data the last presented solution – FBGENMAC, in this blog would be the best. Simply because it can be switched on/off when needed, requires only a single parameter in the user configuration and a few lines of code in the function module we want to use (of course not every standard one have it so if that is the case we will probably still look at the second method). Easy, quick and reliable what would you want more?
